Docker commands in practice
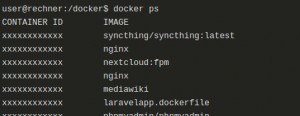
Docker containers can be started, updated and managed using terminal commands. In the post I note commands that I have needed so far for Docker and corresponding examples:
Start container
A Docker container can be started with a simple "run" command:
docker run -d \
--name=testcontainer \
-p=83:80 \
--restart=always \
-v mytestvol:/app \
nginx:latest
The -p 83:80 parameter publishes port 83 and redirects it to port 80 in the container. Our just created container can be accessed in the browser using the IP address of the host and port 83:
Volumes
A volume is a folder on the host operating system to permanently store data of the container. The -v parameter creates a volume and mounts it in the container.
-v mytestvol:/app \
The location for the volume is managed by Docker by default and can be viewed with "docker inspect container name":
docker inspect testcontainer
...
"Mounts": [
{
"Type": "volume",
"Name": "mytestvol",
"Source": "/var/lib/docker/volumes/mytestvol/_data",
...
If you want to use a specific folder of the host operating system, you can also specify a path instead of the volume name (bind mount):
-v /var/docker/mydockerfolder:/app \
":ro" at the end maps the folder read only
-v /OrdnerNurLesend/:/OrdnerNurLesend:ro
See also: Docker data storage: Docker Volumes vs. Host Folders
Container parameters: Network
The parameter: --net=host connects the network card directly.
As an alternative the parameter
-p=external_port:port_in_container can be used:
-p=83:80 would then be able to be called via the IP of the computer with port 83 and internally in the container port 80 is used.
Additionally, with --network=network_name a specific network previously created with "docker network create" can be used. Containers within a network can be called not only by IP, but also by their name (--name): The name and port of the container are then simply used to establish the connection.
Show container
user@rechner:/docker$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
???????????? syncthing/syncthing:latest "/bin/entrypoint.sh …" 12 minutes ago Up 12 minutes (healthy) festive_chandrasekhar
???????????? nginx "/docker-entrypoint.…" 4 days ago Up 12 minutes 80/tcp nextcloud_webserver
???????????? nextcloud:fpm "/entrypoint.sh php-…" 4 days ago Up 12 minutes 9000/tcp nextcloud_fpm
???????????? nginx "/docker-entrypoint.…" 4 days ago Up 12 minutes 80/tcp laraveldev_webserver
???????????? mediawiki "docker-php-entrypoi…" 4 days ago Up 12 minutes 80/tcp mediawiki
???????????? laravelapp.dockerfile "docker-php-entrypoi…" 4 days ago Up 12 minutes 9000/tcp laravelapp
???????????? phpmyadmin/phpmyadmin "/docker-entrypoint.…" 4 days ago Up 12 minutes 0.0.0.0:8081->80/tcp phpmyadmin
???????????? onlyoffice/documentserver "/bin/sh -c /app/ds/…" 4 days ago Up 12 minutes 80/tcp, 443/tcp onlyoffice
???????????? jrcs/letsencrypt-nginx-proxy-companion "/bin/bash /app/entr…" 4 days ago Up 12 minutes letsencrypt-companion
???????????? mariadb "docker-entrypoint.s…" 4 days ago Up 12 minutes 3306/tcp mediawikidb
???????????? jwilder/nginx-proxy:alpine "/app/docker-entrypo…" 4 days ago Up 12 minutes 0.0.0.0:80->80/tcp, 0.0.0.0:443->443/tcp proxy
???????????? mariadb "docker-entrypoint.s…" 4 days ago Up 12 minutes 3306/tcp db
???????????? mariadb "docker-entrypoint.s…" 4 days ago Up 12 minutes 3306/tcp laraveldb
???????????? redis "redis-server --appe…" 4 days ago Up 12 minutes 0.0.0.0:6379->6379/tcp redis
???????????? laradock_nginx "/bin/bash /opt/star…" 3 months ago Up 11 minutes 0.0.0.0:90->80/tcp, 0.0.0.0:444->443/tcp laradock_nginx_1
???????????? laradock_php-fpm "docker-php-entrypoi…" 3 months ago Up 11 minutes 9000/tcp laradock_php-fpm_1
???????????? laradock_phpmyadmin "/run.sh supervisord…" 3 months ago Up 12 minutes 9000/tcp, 0.0.0.0:8082->80/tcp laradock_phpmyadmin_1
???????????? laradock_workspace "/sbin/my_init" 3 months ago Up 12 minutes 0.0.0.0:2222->22/tcp laradock_workspace_1
???????????? laradock_mariadb "docker-entrypoint.s…" 3 months ago Up 12 minutes 0.0.0.0:3306->3306/tcp laradock_mariadb_1
???????????? laradock_redis "docker-entrypoint.s…" 3 months ago Up 12 minutes 0.0.0.0:6380->6379/tcp laradock_redis_1
Stop container
docker stop containername
stop all containers
docker stop $(docker ps -a -q)
Update container
docker pull IMAGENAME
docker stop CONTAINERNAME
docker rm CONTAINERNAME
docker run CONTAINERNAME -parameters
Update Docker Compose Container
docker-compose pull
docker compose up -d
Remove container
docker rm containername
Show network
user@rechner:/docker$ docker network ls
NETWORK ID NAME DRIVER SCOPE
???????????? docker_default bridge local
???????????? host host local
???????????? laradock_backend bridge local
???????????? laradock_default bridge local
???????????? laradock_frontend bridge local
???????????? nginx-proxy bridge local
???????????? none null local
Show detail of a network
docker network inspect docker_default
Calling the bash of a container
docker exec -it containername /bin/bash
Bash commands inside: mysql, mariadb DB restore
gunzip < '/daten/backup.gz' | docker exec -i laradock_mariadb_1 /usr/bin/mysql -u root --password=password dbname
Copy files from a container to the local file system.
docker cp graylog_graylog_1:/usr/share/graylog/data /var/web/graylog/data
Maintenance
Remove unneeded Docker images
docker image prune -a --force
Prune: delete unused data
docker system prune --volumes -f
Monitor / Status
docker ps
docker ps lists all containers:
root@l2:~# docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
b345697c8b1b portainer/agent:latest "./agent" 3 hours ago Up 3 hours portainer_agent.l8lte8805fugp6xdc9sfizwbi.dru1imtp8l56zhby9863j9hi8
Performance
An overview of CPU / RAM and network is provided by the command docker stats
docker stats
Create image and transfer to a remote server
docker save image:latest | gzip > /var/web/image_web.tar.gz
scp /var/web/image_web.tar.gz root@remoteserver:/var/web/image_web.tar.gz
load on the remote server with:
docker load < /var/web/image_web.tar.gz
Show output of each container
docker logs -f containername
Troubleshooting
Docker stops responding
if, for example, commands like docker ps stop responding, the error can be narrowed down using the dockerd --debug command:
sudo dockerd --debug
INFO[2020-12-27T11:58:52.395359797+01:00] Starting up
failed to start daemon: pid file found, ensure docker is not running or delete /var/run/docker.pid
The file can be deleted with
sudo rm /var/run/docker.pid
failed to start daemon: error while opening volume store metadata database: timeout
I was able to fix Docker using the following commands:
Stop all Docker processes:
ps axf | grep docker | grep -v grep | awk '{print "kill -9 " $1}' | sudo sh
delete Metadata.db
sudo rm /var/lib/docker/volumes/metadata.db
Display the status
systemctl status docker
Reinstall Docker
sudo apt-get remove docker-ce
sudo apt-get install docker-ce

{{percentage}} % positive
